- Selenium Commands Cheat Sheet
- Selenium Cheat Sheet C#
- Selenium Cheat Sheet C#
- Selenium Cheat Sheet Javascript
Selenium WebDriver Cheat Sheet Cheat Sheet by Narayanan Palani (Narayanan Palani) via cheatography.com/32112/cs/9838/ Find element VS Find elements driv ‐ er.f ‐ in ‐ dEl ‐ eme ‐ nt When no match has found(0) throws NoSuch Ele men tEx ‐ ception when 1 match found returns a WebElement instance. Selenium WebDriver with Python Cheat Sheet This blog article lists Python Selenium WebDriver commands which are helpful to automate Web Application Testing. Selenium Cheat Sheet - Ruby. GitHub Gist: instantly share code, notes, and snippets. Oct 08, 2020 Selenium Cheat Sheet. This part of the Selenium tutorial includes the Selenium Cheat Sheet. In this part, you will learn various aspects of Selenium that are possibly asked in interviews. Also, you will have a chance to understand the most important Selenium terminologies.
For Python version the link is here
Install Java
To install Java go to this link
IDE
You can use any text editor. I recommend Eclipse as it is free and have extensive support. For list of popular editors , this are the links
Download Selenium
Download selenium webdriver in this link
Dirty our hands !
Import Selenium
Browsers support (Firefox , Chrome , Internet Explorer, Edge , Opera)
Driver setup:
Chrome:
System.setProperty('webdriver.chrome.driver', “'Path To chromedriver');
To download: Visit Here
Firefox:
System.setProperty('webdriver.gecko.driver', 'Path To geckodriver');
To download: Visit GitHub
Internet Explorer:
System.setProperty('webdriver.ie.driver', 'Path To IEDriverServer.exe');
To download: Visit Here
Edge:
System.setProperty('webdriver.edge.driver', 'Path To MicrosoftWebDriver.exe');
To download: Visit Here
Opera:
System.setProperty('webdriver.opera.driver', 'Path To operadriver');

To download: visit GitHub
Browser Arguments:
–headless
To open browser in headless mode. Works in both Chrome and Firefox browser
–start-maximized
To start browser maximized to screen. Requires only for Chrome browser. Firefox by default starts maximized
–incognito
To open private chrome browser
–disable-notifications
To disable notifications, works Only in Chrome browser
Example:
Alternative
Launch URL
Retrieve Browser Details:
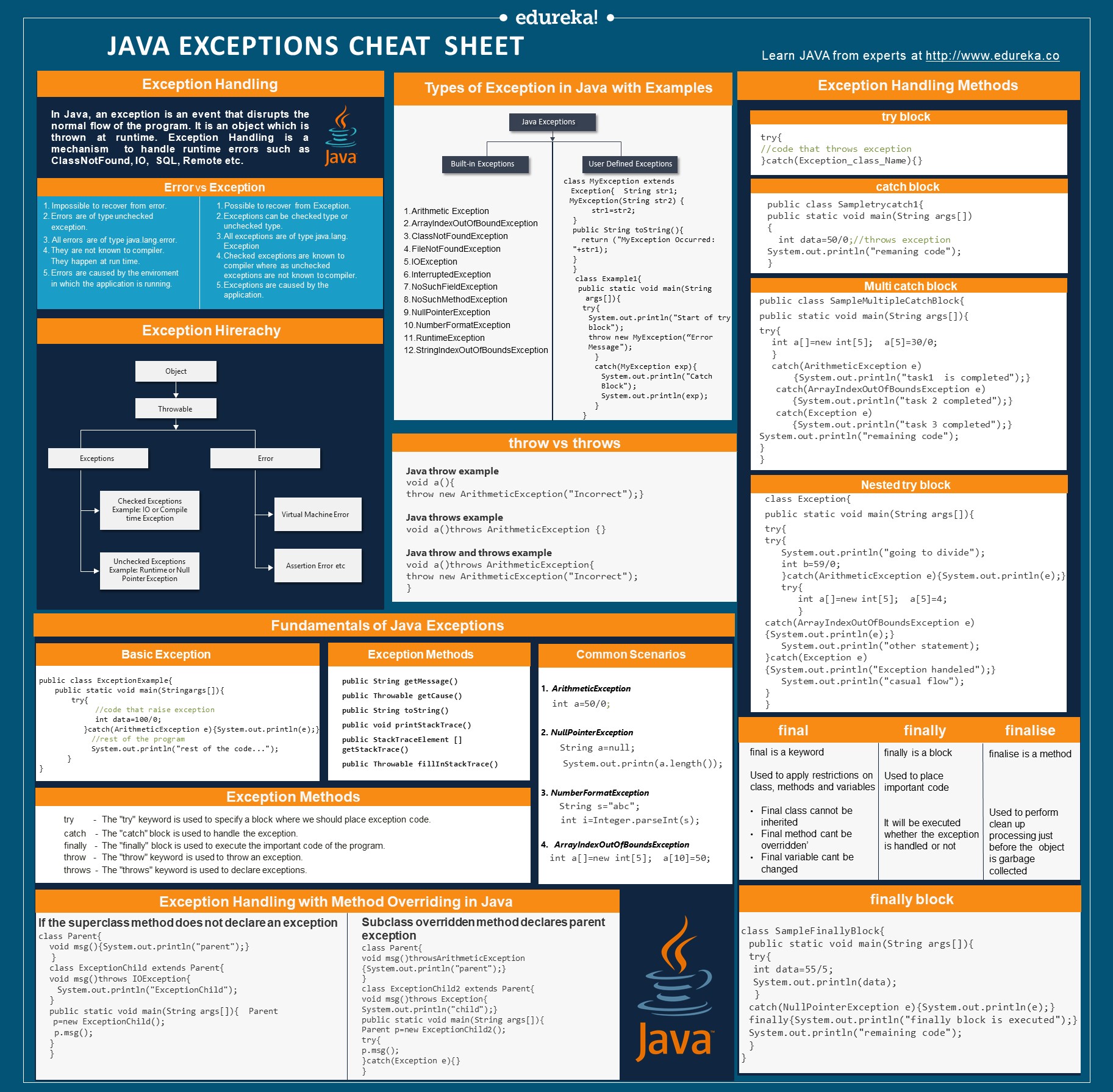
Navigation
Locating Elements
By id
<input id=”login” type=”text” />
By Class Name
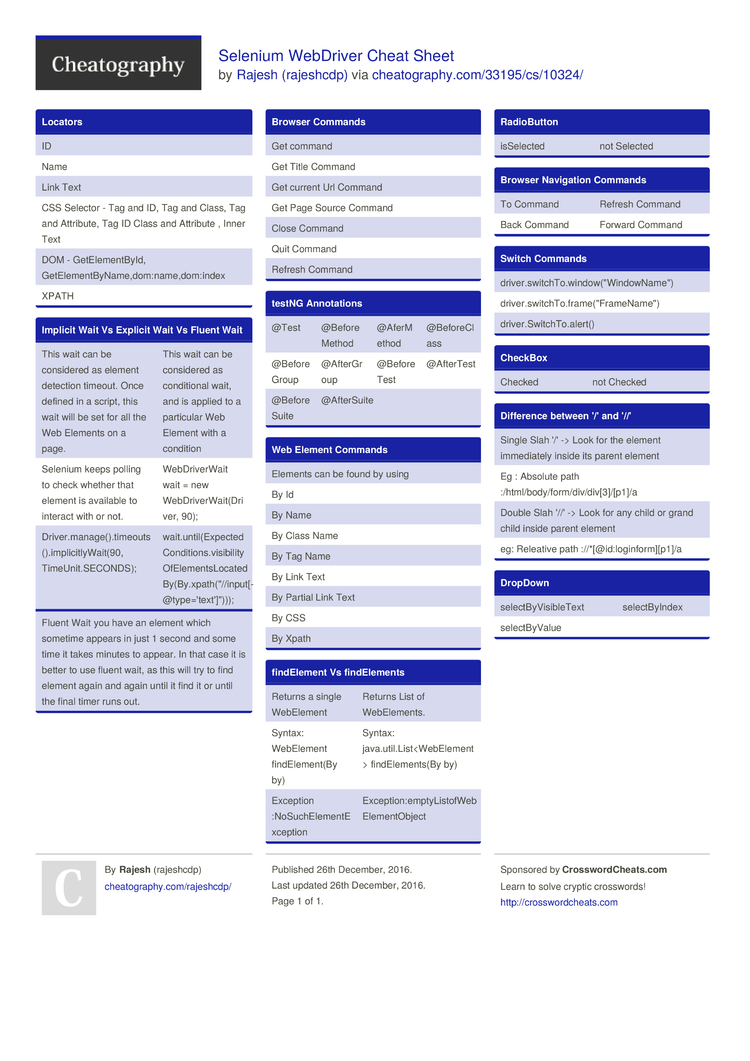
<input class=”gLFyf” type=”text” />
By Name
<input name=”z” type=”text” />
By Tag Name
<div id=”login” >…</div> Db2 dbeaver.
By Link Text
<a href=”#”>News</a>
By XPath
<form id=”login” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
By CSS Selector
<form id=”login” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
Clicking / Input text
Clicking button
Send Text
Waits
Implicit Waits
An implicit wait instructs Selenium WebDriver to poll DOM for a certain amount of time, this time can be specified, when trying to find an element or elements that are not available immediately.
Selenium Commands Cheat Sheet
Explicit Waits Quickbooks desktop for mac 2018 download.
Explicit wait make the webdriver wait until certain conditions are fulfilled . Example of a wait
List of explicit waits
- alertIsPresent()
- elementSelectionStateToBe()
- elementToBeClickable()
- elementToBeSelected()
- frameToBeAvaliableAndSwitchToIt()
- invisibilityOfTheElementLocated()
- invisibilityOfElementWithText()
- presenceOfAllElementsLocatedBy()
- presenceOfElementLocated()
- textToBePresentInElement()
- textToBePresentInElementLocated()
- textToBePresentInElementValue()
- titleIs()
- titleContains()
- visibilityOf()
- visibilityOfAllElements()
- visibilityOfAllElementsLocatedBy()
- visibilityOfElementLocated()
Loading a list of elements like li and selecting one of the element
Read Attribute
Get CSS
CSS values varies on different browser, you may not get same values for all the browser.
Capture Screenshot
This will saved the file as in the path of destFile.
isSelected()
isSelected() method in selenium verifies if an element (such as checkbox) is selected or not. isSelected() method returns a boolean.
Selenium Cheat Sheet C#
isDisplayed()
isDisplayed() method in selenium webdriver verifies and returns a boolean based on the state of the element (such as button) whether it is displayed or not.
isEnabled()
is_enabled() method in selenium python verifies and returns a boolean based on the state of the element (such as button) whether it is enabled or not.
Minimum modules to import
Created : 17 December 2019
Getting Started
Load the Selenium Webdriver library
require 'selenium-webdriver'
Open a browser (Ex: Internet Explorer)
driver = Selenium::WebDriver.for :ie
Go to a specified URL
driver.navigate.to 'http://www.orbitz.com/'

driver.get 'http://www.orbitz.com/'
Close the browser
driver.close
Access an Element
Type somethign in the Text box or text area
driver.find_element(:id,'airOrigin').send_keys('MAA')
To Clear the text from text field driver.find_element(:id,'airOrigin').send_keys [:control, 'a'], :space
Button
driver.find_element(:id,'BUTTON_ID').click
Drop down list
select_box=driver.find_element(:id,'airStartTime')
Selenium Cheat Sheet C#
options=select_box.find_elements(:tag_name=>'option')
options.each do |option_field|
if option_field.text '12a-9a'
option_field.click
break
end
end
Check box
driver.find_element(:id,'airNonStopsPreferred').click
Radio button
driver.find_element(:id,'htlChoice').click
To verify Flights radio button selected or not
driver.find_element(:id,'airChoice').selected?
#if it returns TRUE then radio button already selected.
Return the title of the document
puts driver.title
Selenium Cheat Sheet Javascript
Return true if the specified text appears on the TAG
puts driver.find_element(:class,'welcomeText').text.include?('Welcome to Orbitz')
To Click SPAN Elements
options=driver.find_elements(:tag_name=>'span')
options.each do |span_field|
if span_field.text 'Find Flights'
span_field.click
break
end
end
